먼저 플러터에서 HTTP API를 호출하기 위해 패키지를 설치해야 한다.
flutter pub add http
flutter pub add test
나는 테스트 코드에서 실행시킬 거라 test 패키지도 설치했다. 정확히는 Dart 언어로 Api 호출하는 방법이다.
참고로 아래의 웹 사이트를 이용해 JSON 호출을 할 거다!
JSONPlaceholder - Free Fake REST API
{JSON} Placeholder Free fake API for testing and prototyping. Powered by JSON Server + LowDB. Tested with XV. Serving ~2 billion requests each month.
jsonplaceholder.typicode.com
우리가 GET 해올 데이터이다. 한 번에 됐다면 좋겠다만 프론트는 거의 처음이라 우여곡절이 많다^^...
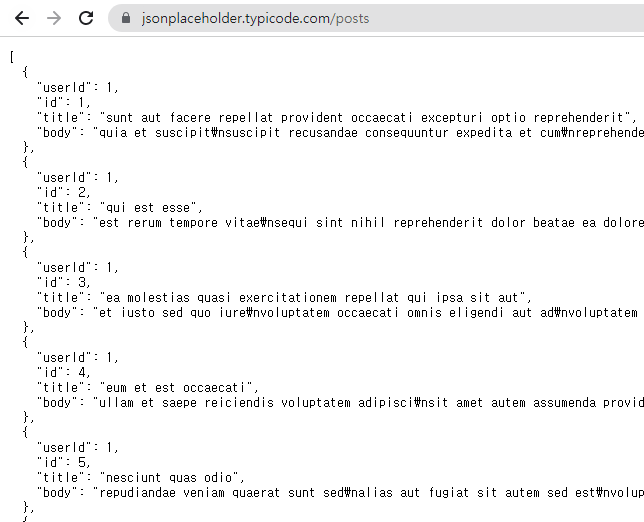
불러온 JSON을 저장할 클래스는 다음과 같다.
class Info {
final int userId;
final int id;
final String title;
final String body;
Info({
required this.userId,
required this.id,
required this.title,
required this.body,
});
factory Info.fromJson(Map<String, dynamic> json){
return Info(
id: json["id"],
userId: json["userId"],
title: json["title"],
body: json["body"],
);
}
}
Dart 문법이 생소하다. field와 constructor, factory 패턴을 사용한 static?? 메서드인 것 같다. 특이하게 생성자를 만들지 않으면 오류가 난다.
Info tmp = Info.fromJson({
"id":1,
"userId":2,
"title":"title",
"completed":false });
위처럼 코드를 작성했을 때 Info 인스턴스가 생성된다. 모르겠으면 외우자. 물론 new 키워드로 생성자 사용해도 된다.
import 'dart:convert';
import 'dart:io';
import 'package:http/testing.dart';
import 'package:test/test.dart';
import 'package:http/http.dart' as http;
import 'Info.dart';
void main() {
final String URL = "https://jsonplaceholder.typicode.com/";
final request = Uri.parse(URL+"posts");
Future<dynamic> fetch() async {
final response = await http.get(request);
print(jsonDecode(response.body));
}
test("API GET 테스트", () async {
await fetch();
});
}
간단한 호출 테스트 코드는 성공하는 것을 확인했다.
이제 Info 클래스로 매핑을 해보자.
간단히 출력 확인하고자 Info 클래스에 메서드를 추가했다.
printing(){
print("userId : ${this.userId}, id : ${this.id}, title : ${this.title}, body : ${this.body}\n");
}
import 'dart:convert';
import 'dart:io';
import 'package:http/testing.dart';
import 'package:test/test.dart';
import 'package:http/http.dart' as http;
import 'Info.dart';
void main() {
final String URL = "https://jsonplaceholder.typicode.com/";
final request = Uri.parse(URL+"posts");
fetch() async {
final response = await http.get(request);
if(response.statusCode==200){
return jsonDecode(response.body)
.map<Info>((json) =>
Info.fromJson(json)).toList();
}
}
test("API GET 테스트", () async {
print("받아오는 중");
await fetch()
.then((value) {
for(var v in value){
v.printing();
}
})
.catchError((error)=>print("error"));
print("받아옴");
});
}
Info로 매핑 후 리스트로 반환하는 테스트 코드이다.
시작부터 끝까지 정상적으로 출력이 된다!
다음 API 호출은 비즈니스 코드에 적용하겠다. await와 async 더 공부해봐야겠다. 그전에 백엔드 api 완성하자!
점점 플러터 공부 프로젝트로 바뀌는 것 같다.
'Project > 모면' 카테고리의 다른 글
도망친 곳엔 낙원은 없다 (0) | 2023.03.29 |
---|---|
[Spring Boot] Security 없이 OAuth2로 Google 로그인 구현, 유저 정보 얻기 (2) | 2023.03.27 |
[Dart] dart 기본 | 자료형, JSON, stream (0) | 2023.03.25 |
[UI/UX] 디자인 레퍼런스 사이트 (0) | 2023.03.21 |
[Flutter] 기본 사용법 간단 정리 (0) | 2023.03.19 |